React
Last updated 1 month and 27 days ago - September 3, 2023
Start here
Free React courses
- Introduction to React by Jack Herrington
- Academind - React Crash Course
- Brad Schiff (LearnWebCode) - React For The Rest Of Us (only the basics of React)
- React Framework.dev - different React resources (books, videos, courses, podcasts) and more
Kent C. Dodds - Free Workshops from Epic React course (free)
JavaScript Version
- React Fundamentals
- React Hooks
- Advanced React Hooks
- Advanced React Patterns
- React Performance
- Testing React Apps
- React Suspense
- Build an Epic React App (Bookshelf)
TypeScript Version (recommended)
Paid React courses
- Academind - React - The Complete Guide (incl Hooks, React Router, Redux)
- Brad Schiff (LearnWebCode) - React For The Rest Of Us
- Kent C. Dodds - Epic React (if you need the video format + solutions + bonus content)
Additional content
What to know before react
- Brad Schiff (LearnWebCode) - What is React and what problems does it solve? (Vanilla JS vs React comparison)
- Kent C. Dodds. - JavaScript to Know for React
- Web Dev Simplified - Do You Know Enough JavaScript To Learn React
- How well should I know React before applying to my first job?
Common mistakes
- Jack Herrington - 3 React Mistakes, 1 App Killer
- Jack Herrington - Stop Writing Fake React Code
- Josh W. Comeau - Common Beginner Mistakes with React
- Stop using SVG-in-JS (bundle size issues)
Hooks
About useEffect hook
- Jack Herrington - React 18: useEffect Double Call; Mistake or Awesome?
- Jack Herrington - Mastering React’s useEffect
- Jack Herrington - Common React Mistakes: useEffect - Part 1
- Jack Herrington - Common React Mistakes: useEffect - Part 2
- React Docs - You Might Not Need an Effect (when to use the useEffect hook)
- David Khourshid - Goodbye, useEffect (how to use useEffect hook properly)
- Jack Herrington - Mastering useEffect: Beware of depending on values you set
- Jack Herrington - Mastering useEffect: What Goes In Dependency Arrays
About useState hook
- Jack Herrington - useState: Asynchronous or what?
- A cure for React useState hell - Steve Sewell on dev.to
Custom hooks
- useHooks - Easy to understand React Hook recipes by ui.dev
- useHooks-ts - custom React hooks written in TypeScript
- Felix Gerschau - Form validation with React Hooks WITHOUT a library: The Complete Guide
- Web Dev Junkie - How you can use custom hooks to abstract your React code
- TomDoesTech - You Need to Know This React Design pattern
- React Hook for Avoiding Flash of Empty UI While Data Transitions
React + TypeScript
- Matt Pocock - Learn React with TypeScript
- Jack Herrington - Mastering React Hooks with TypeScript
- React TypeScript Cheetsheets
- React
ComponentProps<T>
Type Helper - Matt Pocock - Fix your useRefs with ElementRef
Folder structure
- What’s the best component / folder architecture for a project to scale?
- Josh W. Comeau - Delightful React File/Directory Structure
- Screaming Architecture - Evolution of a React folder structure
- Richard Oliver Bray - How to structure files in large React projects
- Tania Rascia - React Architecture: How to Structure and Organize a React Application
- Web Dev Simplified - How To Organize React Projects
- Theo - ping.gg - Your Folder Structure Hurt Me (reaction to Web Dev Simplified’s folder structure video + from 11:06 shows and explain folder structure in his projects)
- React project structure for scale: decomposition, layers and hierarchy
- Project Standards for High-Quality Code - React Handbook
Principles for React
- Traversy Media - 5 Pro-Level React Do’s & Don’ts with Jack Herrington
- Alex Kondov - Tao of React
- Web Dev Junkie - Refactoring and decoupling some React code (DRY, KISS, YAGNI, WET)
- Fireship - 10 React Antipatterns to Avoid - Code This, Not That!
- React Philosophies - what you need to know, when writing the React code?
- Applying SOLID principles in React
- Kent C. Dodds - Inversion of Control
- AppUnite - Vue’s Slots Composition adapted to React - how and when to use it
- Tips for Writing Better React Code
- Kent C. Dodds - Stop using isLoading booleans
Principles for React in Polish
- Michał Taszycki - Architektura aplikacji w React.js – czyli poszukiwania Świętego Graala.
- Krzysztof Jendrzyca - The Zen of React (jak utrzymywać czysty kod w React)
- Michał Taszycki - Legacy React - czyli jak zrobić refactoring i nie stracić pracy!
Przeprogramowani - mini-seria ze wzorca State Machine
Przeprogramowani - Jak NIE zarządzać stanem komponentów? | Wzorzec State Machine #1
Przeprogramowani - Jak zastosować State Machine w praktyce? | Wzorzec State Machine #2
Przeprogramowani - Zarządzanie stanem z XState | Wzorzec State Machine #3
Warte zobaczenia, bo daje szerszy obraz na to, jak można efektywniej zarządzać stanem w React. Trwa około 40 minut.
Slajdy z powyższego wykładu po stronie trzeba poruszać się strzałkami (lewo/prawo).
Fetching data in React
Advices from Dan Abramov (member of React Core Team)
Screenshots
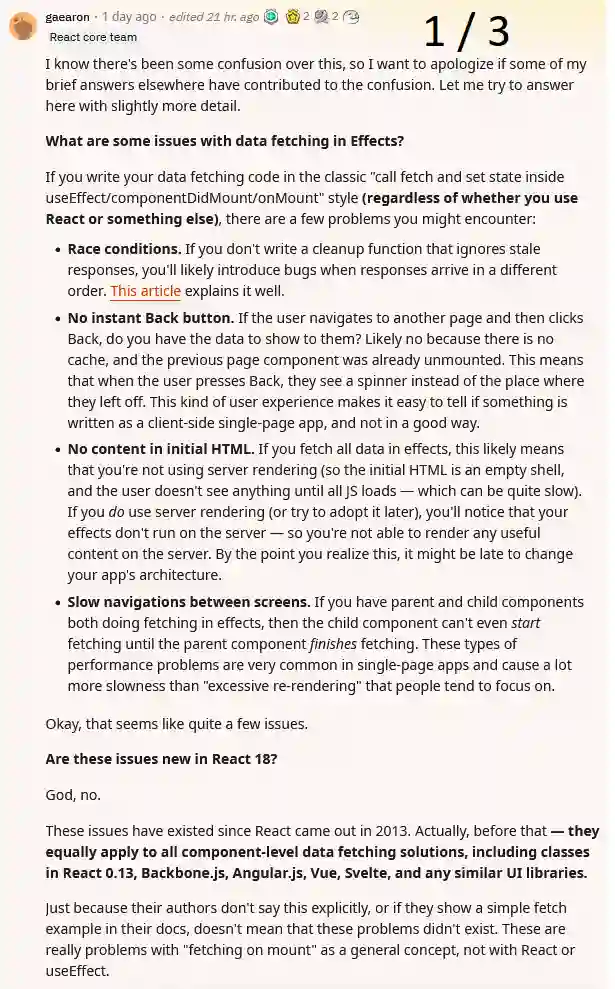
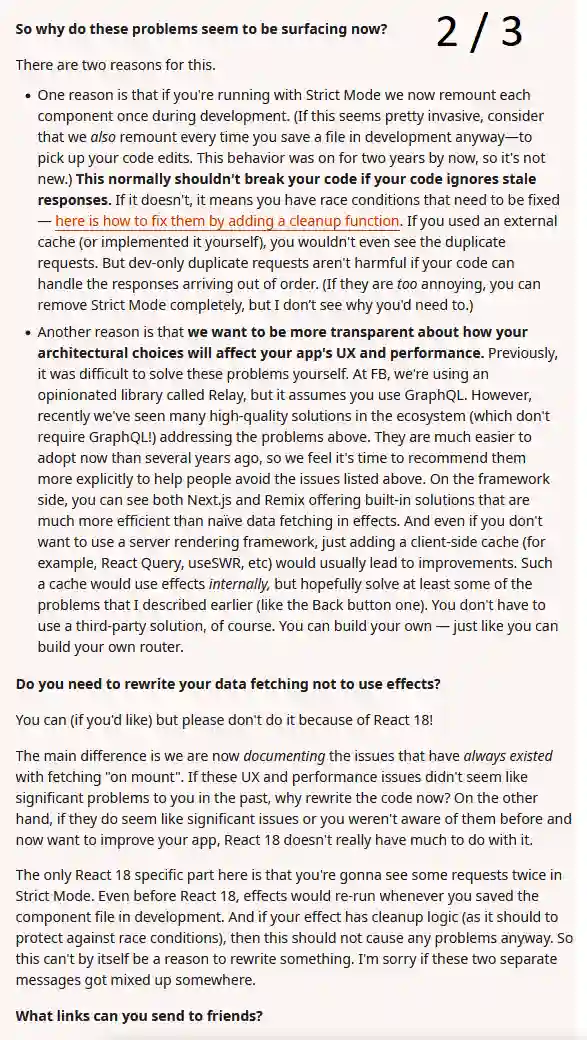
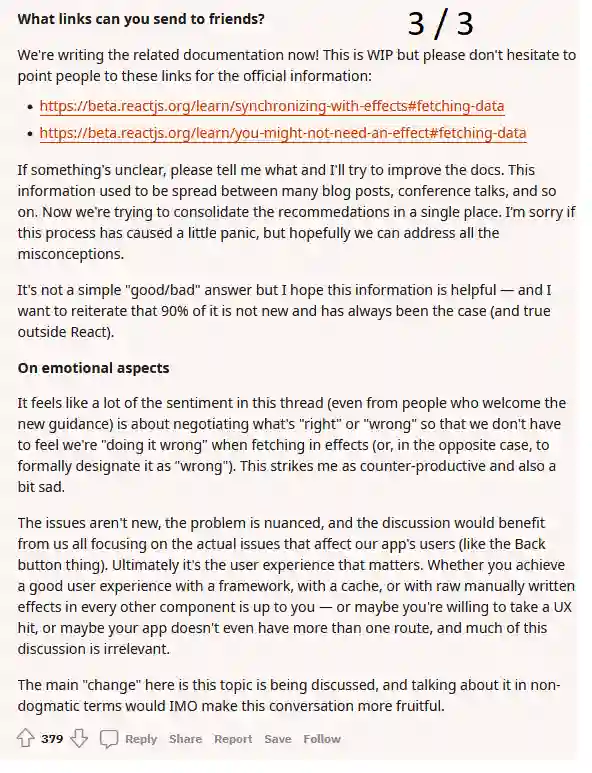
React Context
State Management
- Jack Herrington - React State Management – Intermediate JavaScript Course
- Theo - ping.gg - You still use Redux? (how to pick the right state management tool?)
- Redux - bewebdev.tech
- Web Dev Simplified - learn React Query
- Web Dev Simplified - learn XState (This Library Makes State Management So Much Easier)
React Portals
- Theo - ping.gg - how to use React Portals and why?
- Teleportation in React: Positioning, Stacking Context, and Portals
Error handling
React testing
- Jack Herrington - TypeScript/React Testing: Components, Hooks, Custom Hooks, Redux and Zustand
- More about React testing in our dedicated docs section - bewebdev.tech
Performance in React
- Web Dev Simplified - How To Maximize Performance In Your React Apps
- Web Dev Simplified - Speed Up Your React Apps With Code Splitting
- Jack Herrington - Mastering React Batch Updating
- React is slow, what now?
- Lydia Hallie - Advanced Rendering Patterns (what shortcuts like ISR, SSG and SSR really mean? )
- Optimizing performance in a React application
- Modus - How to optimize a React.js app bundle performance to load under 3s
Memoization in React
useCallback and useMemo
- Kent C. Dodds - When to useMemo and useCallback.
- Demystifying React Hooks: useCallback and useMemo
- Web Dev Simplified - Learn useMemo In 10 Minutes
- Web Dev Simplified- Learn useCallback In 8 Minutes
React.memo
React libraries
Debugging React apps
- Bartosz Szczeciński - Intro to debugging ReactJS applications
- Web Dev Junkie - Tips and Tricks for Debugging React Applications
- Kent C. Dodds - React DevTools
- How To Debug React Components Using React Developer Tools
How React works under the hood
- Reconciliation - React docs
- What is Diffing Algorithm? - geeksforgeeks
- Virtual DOM and Internals
- React fiber architecture
- How does React work – deep dive into the React framework - The Software House
- The Interactive Guide to Rendering in React
WebSockets
Animations
React-Spring
Framer Motion
- The introduction of Framer Motion
- CodeSnap - Framer Motion | Page Transitions in React
- Fireship - Springy Animated Modals // Framer Motion & React Tutorial for Beginners
- PedroTech - Animations In React - Framer-Motion Tutorial
Polish recruitment materials
Other React materials
- Web Dev Simplified - Most Senior React Devs Don’t Know How To Fix This
- Jack Herrington - Don’t Follow THIS Bad React Advice!
- Web Dev Junkie - How to implement the MVC pattern in React (very interesting approach to this problem)
- Web Dev Junkie - Applying layered architecture to my t3 stack api
- uidotdev - The Story of React
- uidotdev - The Story of Concurrent React
- Jack Herrington - React UI Library Structure, Storybook and Tests
- Jack Herrington - Rendering React Components Conditionally